Websocket Interface
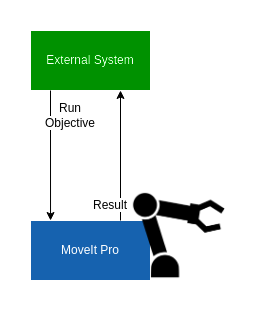
After you have built an application with MoveIt Pro you’ll need to integrate it with a higher layer system. How you do that depends on the tech stack of your larger system. We’ve chosen interfaces that should work with most existing systems. Some examples are:
- ROS + Python or C++
- Websocket + Python, JavaScript, Rust, or Java
In applications where you do not need to interact with the UI, you can start MoveIt Pro headless.
This can be done by passing the --headless
argument to the MoveIt Pro application on startup:
./moveit_pro run -c <your_robot_config_name> --headless
Note
If your software uses ROS, we’ve built a library to make it easy to externally execute Objectives using the MoveIt Pro SDK. Read the Python API tutorial to learn more.
We use the Rosbridge Protocol to communicate between the Runtime and UI. If you are building a system that needs to interact with MoveIt Pro but does not need the complexity of depending on ROS you too can use the websocket interface. There are libraries for many popular modern languages that implement the rosbridge protocol:
MoveIt Pro Runtime runs a rosbridge server listening on port 3201
.
To connect to it across your network expose that port in your Robot’s firewall settings.
To start an Objective you only need to know its name. This can be found in the UI here:
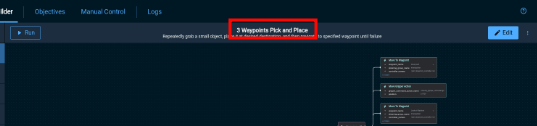
The service topic MoveIt Pro can take requests on is /execute_objective
.
For example, to communicate with MoveIt Pro from a Python script you can install the roslibpy library:
pip install roslibpy
Here is an example Python script that will start the “Close Gripper” Objective, wait for the response, and then print the response. Note that you will need to know the IP address of your robot if not running on the same machine.
import roslibpy
# Connect a client to the rosbridge running on the robot
# Replace the host with the IP of your robot
client = roslibpy.Ros(host='0.0.0.0', port=3201)
client.run()
# Setup the execute objective service
execute_objective = roslibpy.Service(
client,
'/execute_objective',
'moveit_studio_sdk_msgs/srv/ExecuteObjective'
)
# Create a request to run the objective
request = roslibpy.ServiceRequest({"objective_name": "Close Gripper"})
# Call the service and print the response
response = execute_objective.call(request)
print(response)
This interface can be used for much more than just triggering Objectives. Through the rosbridge protocol you have access to the full ROS messaging system inside MoveIt Pro. Some examples of projects you could build using this interface:
- Webapp UI
- Machine Learning Image Pipeline
- Multi-Robot Coordination
- Factory Controller
- Mobile App
Websockets are useful when you need to interact with a ROS based robot system across a network. When you use the rosbridge protocol, you are giving up some message latency and throughput performance in exchange for not sending ROS discovery traffic across your network. There are solutions for bridging multiple ROS networks across a larger networks also if that is preferred. However, in many cases using the rosbridge protocol makes your non-ROS application easier to develop and deploy.